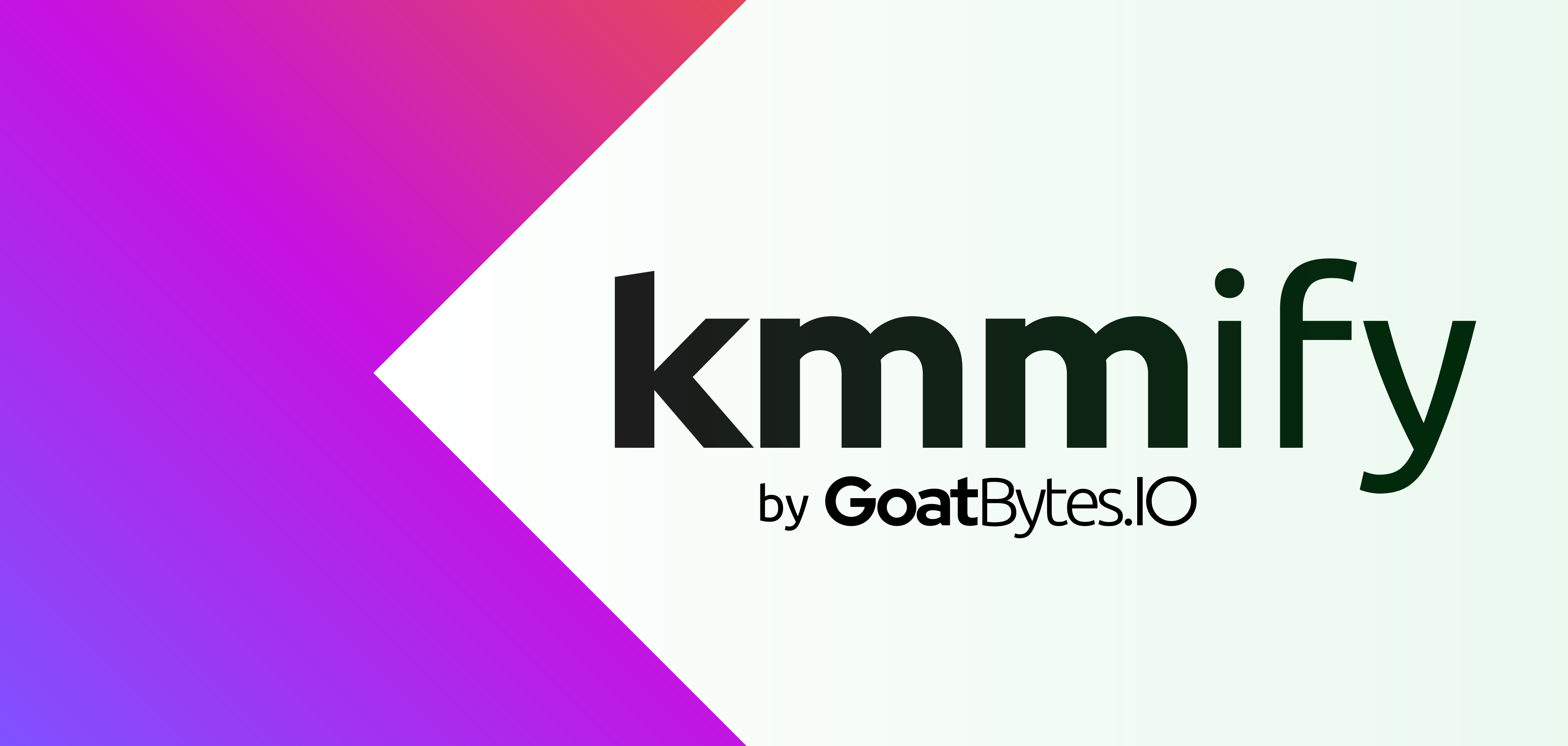
Natural Language Processing (NLP) is a subfield of Artificial Intelligence (AI) that deals with the interaction between humans and computers using natural language. It is an essential component of modern mobile applications that enable users to communicate with mobile devices using voice commands or text input. In this article, we will discuss the current state of natural language processing on mobile devices, compare different solutions, including Google Dialogflow, ChatGPT, and open-source alternatives, and provide some code examples using Kotlin.
Google Dialogflow is a cloud-based NLP service that enables developers to build conversational interfaces for various platforms, including mobile devices. It uses machine learning algorithms to understand natural language queries and provides responses to users in a conversational format. Dialogflow supports various languages and provides a rich set of tools and APIs for developers to build custom chatbots and voice assistants. Dialogflow can be integrated with Google Assistant, Slack, Facebook Messenger, and other platforms.
To use Dialogflow in a mobile app, developers need to create a Dialogflow agent that defines the conversation flow and handles user queries. The agent can be created using the Dialogflow console, which provides a graphical interface for designing conversation flows and training the NLP model. Once the agent is created, developers can use the Dialogflow API to send user queries and receive responses from the NLP model.
Here is an example of using Dialogflow in a Kotlin app:
val credentials = GoogleCredentials.fromStream(FileInputStream("path/to/credentials.json"))
val projectId = "my-project-id"
val settingsBuilder = SessionsSettings.newBuilder()
.setCredentialsProvider(FixedCredentialsProvider.create(credentials))
.build()
val sessionsClient = SessionsClient.create(settingsBuilder)
val sessionName = SessionName.of(projectId, UUID.randomUUID().toString())
val queryInput = QueryInput.newBuilder()
.setText(TextInput.newBuilder().setText("What's the weather like today?").build())
.build()
val detectIntentRequest = DetectIntentRequest.newBuilder()
.setSession(sessionName.toString())
.setQueryInput(queryInput)
.build()
val response = sessionsClient.detectIntent(detectIntentRequest)
This code sends a user query to Dialogflow and receives a response from the NLP model. The response can be used to generate a chatbot or voice assistant response.
ChatGPT is a language model developed by OpenAI that can generate natural language responses to user queries. It is a powerful tool for building chatbots and voice assistants that can understand complex user queries and provide accurate responses. ChatGPT is available as a cloud-based API and can be integrated into mobile apps using HTTP requests.
To use ChatGPT in a Kotlin app, developers need to send user queries to the ChatGPT API and receive responses in JSON format. Here is an example code snippet:
val client = OkHttpClient()
val url = "https://api.openai.com/v1/engines/davinci-codex/completions"
val requestBody = RequestBody.create(
MediaType.parse("application/json"),
"""
{
"prompt": "What's the weather like today?",
"max_tokens": 50,
"temperature": 0.7
}
""".trimIndent()
)
val request = Request.Builder()
.url(url)
.addHeader("Authorization", "Bearer $API_KEY")
.post(requestBody)
.build()
val response = client.newCall(request).execute()
val jsonResponse = JSONObject(response.body()?.string())
val outputText = jsonResponse.getJSONArray("choices")
.getJSONObject(0)
.getString("text")
This code sends a user query to the ChatGPT API and receives a response in JSON format. The response contains a list of possible completions of the user's query, and we extract the text of the first completion as the output of our chatbot or voice assistant.
There are several open-source NLP libraries and frameworks available for mobile app development. Some of the popular ones are:
Natural Language Processing is an essential component of modern mobile applications that enables users to communicate with mobile devices using natural language. In this article, we discussed the current state of natural language processing on mobile devices and compared different solutions, including Google Dialogflow, ChatGPT, and open-source alternatives. We also provided some code examples using Kotlin to illustrate how to use these solutions in mobile app development. Developers can choose the best solution based on their requirements and resources to build powerful chatbots and voice assistants for their mobile apps.