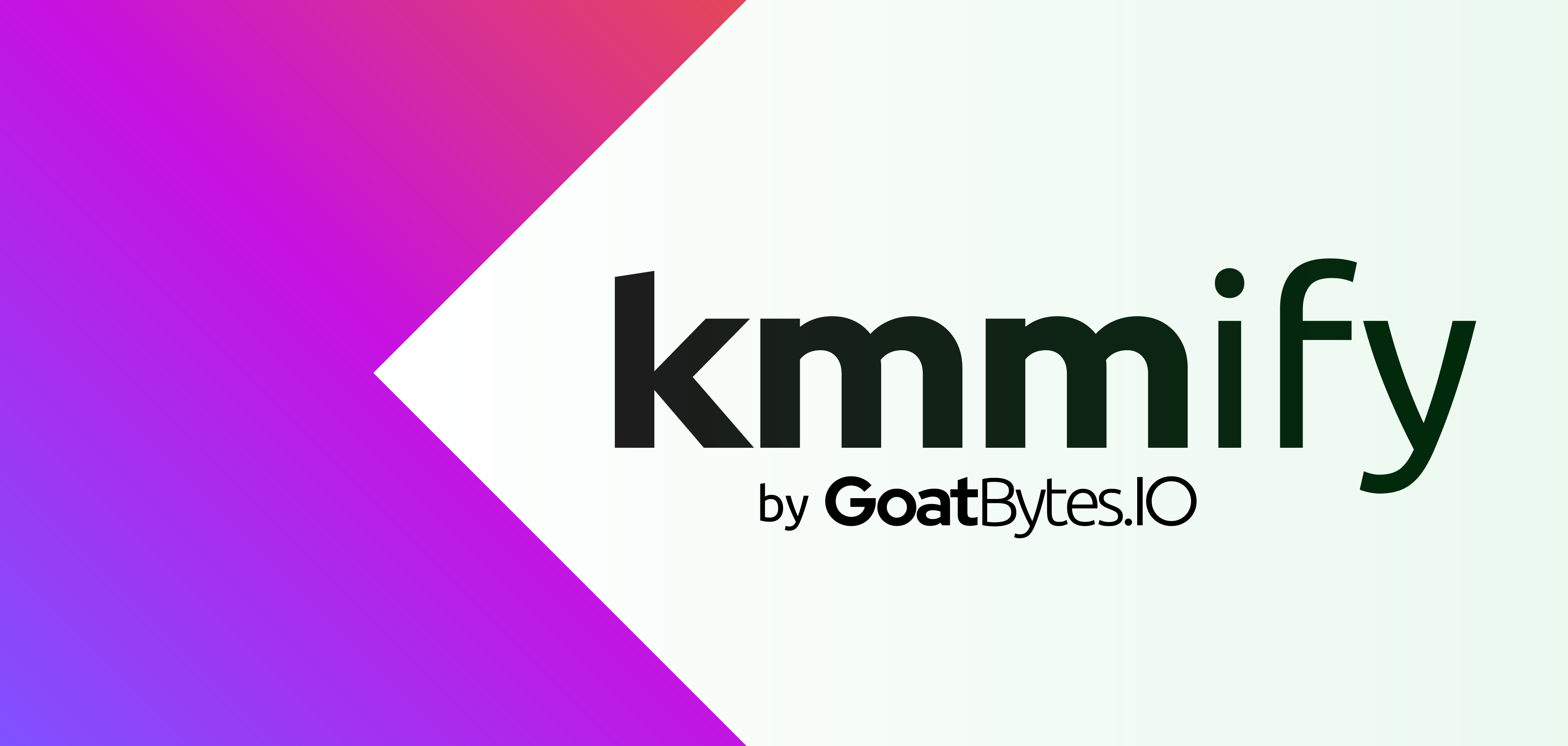
In the ever-evolving landscape of Android development, Jetpack Compose is the beacon of hope for those seeking to escape the verbose and cumbersome XML layouts. It introduces a paradigm shift from the imperative world to a more intuitive, declarative UI approach. But with great power comes great responsibility, especially when it comes to managing state and understanding recomposition.
Imagine writing the script for a play where the scenes change dynamically based on the audience’s reactions. This is essentially what managing state in Jetpack Compose looks like. State in Compose dictates the UI’s appearance and behavior, making its management a cornerstone of Compose architecture.
In the simplest terms, state refers to any data that, when altered, should trigger the UI to update. Think of it as the mood of your cat 😼 that changes from “judgmentally staring” to “furiously ignoring” when you stop petting.
To tame this feline-like unpredictability of UI state, we employ techniques like state hoisting
and the remember
function.
State hoisting is akin to moving the cat’s food bowl higher where it can’t randomly decide to knock it over. By elevating state to a common ancestor, we centralize state management, making our composables purer and UI updates more predictable.
Using remember
in Compose ensures that your UI remembers the state through recompositions, much
like how your cat never forgets the sound of the treat bag rustling.
To illustrate these concepts, let’s dive into an example: a “Cat Mood Detector” app. This app, determines your cat’s mood based on the pampering it receives.
@Composable
fun CatMoodDetector() {
var pamperingScore by remember { mutableStateOf(0) } // Remembering the pampering score
Column(horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.padding(16.dp)) {
Text(text = "Cat Mood Detector", style = MaterialTheme.typography.h4)
MoodDisplay(pamperingScore) // Mood based on pampering score
// Interface to adjust the pampering score
Row {
Button(onClick = { if (pamperingScore > 0) pamperingScore-- }) {
Text("-")
}
Button(onClick = { pamperingScore++ }) {
Text("+")
}
}
Text(text = "Pampering Score: $pamperingScore", style = MaterialTheme.typography.subtitle1)
}
}
This snippet showcases state hoisting (pamperingScore
is managed by CatMoodDetector
) and the use
of remember
to persist state across recompositions.
@Composable
fun MoodDisplay(pamperingScore: Int) {
val mood = when {
pamperingScore > 10 -> "Ecstatic 😺"
pamperingScore > 5 -> "Happy 😸"
pamperingScore > 0 -> "Meh 😼"
else -> "Grumpy 😾"
}
Text(text = "Mood: $mood", style = MaterialTheme.typography.h5, modifier = Modifier.padding(8.dp))
}
MoodDisplay
, a stateless composable, reacts to pamperingScore
changes, demonstrating scoped
recomposition where only components observing state changes get updated.
Recomposition in Compose is like your cat deciding to relocate from the sunny spot on the rug to the top of your laptop during an important meeting—it happens when it’s least expected but is a natural response to the environment.
Efficient recomposition requires understanding which components need to be redrawn upon state changes and optimizing accordingly to prevent the entire UI from being unnecessarily updated.
Jetpack Compose is a powerful tool that simplifies UI development, but mastering state management and recomposition is crucial for harnessing its full potential. By understanding these core concepts, you can create delightful UIs that respond intuitively to user interactions, much like your cat’s mood changes with your pampering. 🐱